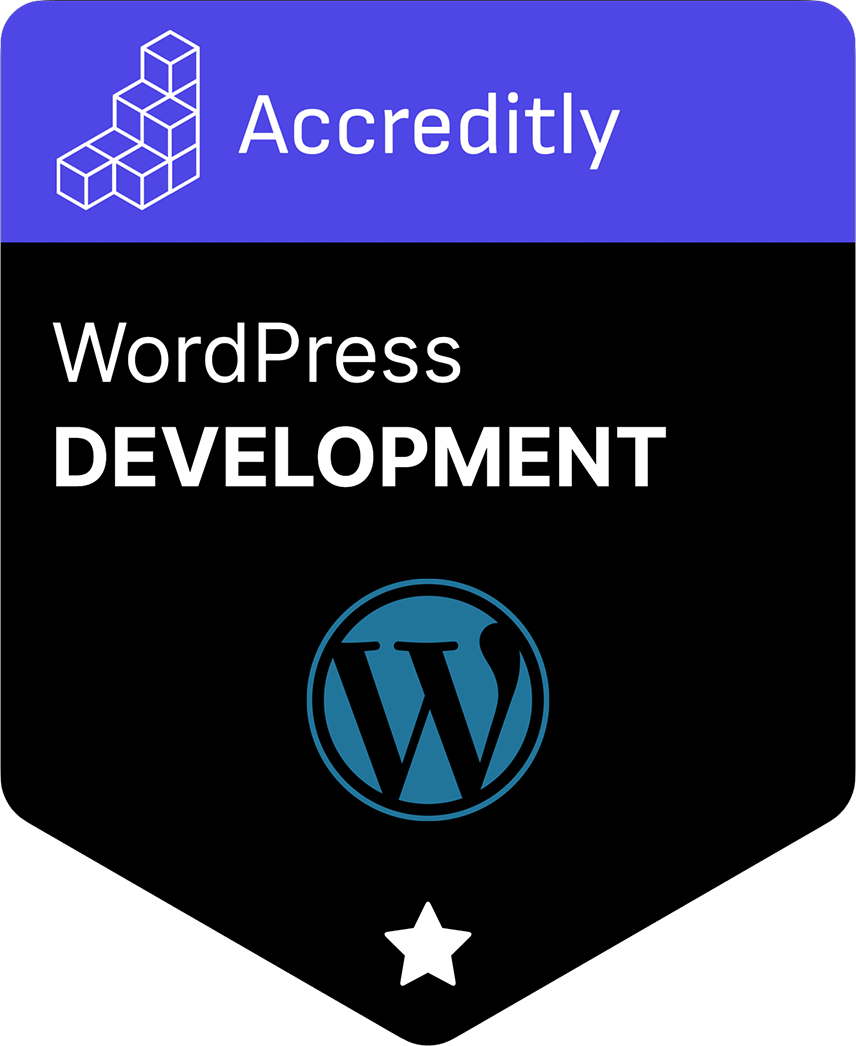
How to Access Query Parameters in WordPress: Methods and Best Practices
Query parameters are an integral part of web development, especially when it comes to dynamic sites like those built on WordPress. They appear in URLs after a question mark (?) and are used to pass data between pages, influencing how a site behaves based on user input. Understanding how to effectively access these parameters can significantly enhance the functionality of your WordPress site. In this article, we will explore various methods for accessing query parameters in WordPress.
What are Query Parameters?
A query parameter is a key-value pair in a URL that allows for the transmission of data. For example, in the URL https://example.com/page?param1=value1¶m2=value2
, param1
and param2
are the query parameters, each associated with specific values.
Methods to Access Query Parameters
Method 1: Using get_query_var()
If you have custom query variables added via WordPress rewrites or are working within a WordPress loop, the get_query_var()
function is your go-to tool. First, you need to register your custom query variable:
function add_query_vars_filter($vars) {
$vars[] = "my_param";
return $vars;
}
add_filter('query_vars', 'add_query_vars_filter');
Then, retrieve the value like this:
$my_param = get_query_var('my_param');
Method 2: Accessing Standard Query Parameters
For standard query parameters, you can utilize the global $_GET
array:
$param1 = isset($_GET['param1']) ? $_GET['param1'] : '';
Method 3: Using the WP_Query
Object
When working within a WordPress loop, you can directly access query parameters from the WP_Query
object:
global $wp_query;
$param1 = $wp_query->query_vars['param1'] ?? '';
Method 4: Using filter_input()
For a more secure approach, consider using the filter_input()
function, which allows for input sanitization:
$param1 = filter_input(INPUT_GET, 'param1', FILTER_SANITIZE_STRING);
Best Practices for Handling Query Parameters
- Validation and Sanitization: Always validate and sanitize query parameters to mitigate security risks.
- Check for Existence: Verify that a query parameter exists before using it to avoid undefined index errors.
- Utilize WordPress Functions: Make use of WordPress-specific functions whenever possible, as they often handle common issues seamlessly.
Use Cases for Query Parameters
Query parameters can serve a variety of purposes, including:
- Filtering Posts: Display posts based on specific criteria, such as category or date range.
- Search Results: Manage search queries and present results effectively.
- Page Navigation: Control pagination for posts or custom post types.
By understanding and utilizing query parameters, you can significantly improve the interactivity and user experience of your WordPress site.